AWS CDK (Cloud Development Kit) lets you define your cloud infrastructure using programming languages like TypeScript, Python, or Go instead of writing/editing JSON or YAML templates for CloudFormation.
Why use AWS CDK?
Manually setting up cloud resources in the AWS console can be repetitive and error-prone. AWS CDK lets you automate these tasks using your favorite (or at least most used) programming language, making your workflows faster and more reliable.
CDK is like an abstraction from CloudFormation. Once you’ve defined and configured your infrastructure, the cdk
will compile your code into CloudFormation templates and then provision those resources onto your AWS account.
AWS CDK is more developer friendly. It allows developers to use and share components easily, making it more maintainable, and it leverages IDE features like autocompletion and syntax checking.
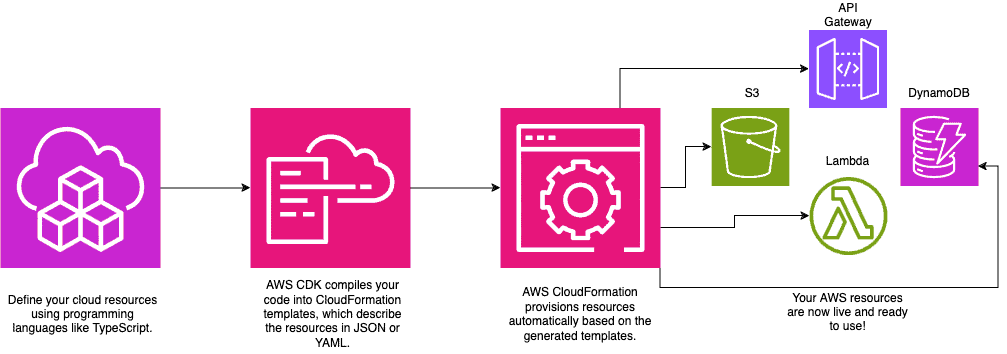
AWS CDK and AWS SDK: What’s the Difference?
Try not to confuse the CDK with the Software Development Kit (SDK) π.
While both AWS CDK and AWS SDK allow you to interact with AWS resources, their purposes differ significantly:
- AWS SDK is used to make API calls to interact with AWS resources at runtime. For example, uploading a GeoJSON file to an S3 bucket.
- AWS CDK defines and provisions infrastructure (e.g. creating an S3 bucket).
Sure, you can create resources with the sdk
. For example, this is how you’d go about creating an S3 bucket:
// Written in TypeScript
import { S3 } from 'aws-sdk';
const s3 = new S3();
const createBucket = async () => {
const bucketName = 'my-sdk-bucket';
try {
const response = await s3.createBucket({ Bucket: bucketName }).promise();
console.log(`Bucket created successfully: ${response.Location}`);
} catch (error) {
console.error('Error creating bucket:', error);
}
};
createBucket();
But the cdk
is better suited for managing and maintaining infrastructure as code.
We’d use the cdk
to create an S3 bucket as part of the infrastructure while the sdk
would perform operations like uploading, downloading, or deleting files on that same bucket during runtime.
AWS CDK vs CloudFormation: How Are They Related?
As stated before, the cdk
is like an abstraction from CloudFormation. Everything you code up will eventually become a CloudFormation template.
What separates CloudFormation from cdk
is that with CloudFormation you declaratively define resources using JSON or YAML. While with the cdk
you can use a programming language.
βοΈ: An advantage with using the cdk
is instead of manually managing YAML files, you can define reusable constructs in a language of your choices, enabling rapid development and iteration!
Imagine having to yaml-engineer a file with over 2000 lines π. Using CloudFormation can become verbose and repetitive for complex setups.
You’d also have to manually copy and paste YAML or JSON configurations if you want to reuse the same components. CDK constructs are the counterpoint for that issue of manually copying. Constructs are reusable components that use specific properties. Example properties for an S3 construct would be:
- Versioning
- Access Control
- Bucket Encryption
- Bucket Policy
- Logging
- Event Notification
- Removal Policy
- etc.
Not to mention it’s easier to maintain cdk
code. Sure, static files like yaml and json can be versioned but it’s not as intuitive as versioning code and having the ability for your team to do code review on pending changes.
You also can’t test static files. cdk
allows for unit testing.
Lastly, there are prebuilt constructs (either from the AWS CDK construct library or third-party libraries you can find on npmjs) that are ready to use. With CloudFormation, you’d need define those custom configurations manually.
Setting Up AWS CDK
For more detailed instructions, you can follow the AWS CDK Developer Guide or work through this CDK Workshop alongside this post.
Bootstrapping
Bootstrapping is one of the most crucial steps when setting up AWS CDK. It prepares your AWS environment by creating necessary resources for CDK to interact with your AWS environment (environment means account and region).
The resources that are created are:
- an S3 bucket for storing CDK project files
- an ECR for Docker images
- IAM roles for granting permissions needed by the AWS CDK to perform deployments
Without bootstrapping, you wonβt be able to deploy CDK applications.
If you have multiple regions you must run cdk bootstrap
even if the account number is the same. This is because CloudFormation is a regional service, so the resources it provisions and manages are scoped to a specific AWS region.
Install AWS CDK
We’re working with CDK v2.
npm install -g aws-cdk
Bootstrap Environment (One-time setup)
cdk bootstrap aws://<account-id>/<region>
Bootstrapping: Permissions Issues
You might run into permissions issues…I know I have several times when I tried getting started with the CDK. Double check and see if the IAM role you’re using for cdk
deployments has the necessary permissions.
There’s an awesome blog post that goes into the required permissions you’re IAM role needs in order to successfully bootstrap your environment. Compare and contrast your IAM policy/permission. You may need to add to the policy or use another IAM role.
One positive thing to note is that CDK v2 automatically creates and uses its own IAM roles during the bootstrap process, so you donβt need to manually manage permissions for deploying resources. You only need to grant your user or role the permission to assume these CDK-managed roles.
However, in order to utilize the CDK, you must get past the bootstrapping process.
Create a New CDK Project
mkdir my-cdk-app
cd my-cdk-app
cdk init app --language=typescript
Make sure the folder you want to initialize your cdk
project is empty.
cdk init
generates a predefined project structure, including directories, configuration files, and dependencies. If you try to work from a non-empty directory, AWS CDK will refuse to initialize the project to avoid overwriting existing files.
Install AWS Constructs
npm install @aws-cdk/aws-s3 @aws-cdk/aws-lambda
After initializing a CDK app, the following project structure is created:
my-cdk-app/
βββ bin/
β βββ my-cdk-app.ts # Entry point for the CDK application.
βββ lib/
β βββ my-cdk-app-stack.ts # Defines your stack(s) and AWS resources.
βββ test/
β βββ my-cdk-app.test.ts # Unit tests for your CDK application.
βββ node_modules/ # Installed dependencies (autogenerated).
βββ package.json # Project dependencies and scripts.
βββ cdk.json # CDK configuration file.
βββ tsconfig.json # TypeScript configuration.
βββ README.md # Auto-generated readme for your project.
The bin/
directory contains the logic that specifies which stacks to deploy. For example:
import * as cdk from 'aws-cdk-lib';
import { GeoCdkAppStack } from '../lib/my-cdk-app-stack';
const app = new cdk.App();
new GeoCdkAppStack(app, 'MyCdkAppStack');
The lib/
directory contains stack definitions, which define your AWS resources using constructs.
import * as cdk from 'aws-cdk-lib';
import * as s3 from 'aws-cdk-lib/aws-s3';
export class MyCdkAppStack extends cdk.Stack {
constructor(scope: cdk.App, id: string, props?: cdk.StackProps) {
super(scope, id, props);
new s3.Bucket(this, 'MyDataBucket', {
versioned: true,
publicReadAccess: false,
});
}
}
Deploy the Stack
A stack groups together AWS resources. It’s essentially the same thing as a CloudFormation stack.
For example, a stack might include resources like S3 buckets, Lambda functions, and DynamoDB tables that work together.
cdk deploy
Destroying a Stack
In case you want to clean up resources and avoid unnecessary costs, you can destroy your stack.
This command removes all resources provisioned by your stack. Any other resources that weren’t created by your stack will not be removed/modified.
cdk destroy
Takeaways
The initial set up for AWS CDK is steep, but once you’ve crossed that peak you’ll be able to utilize all the amazing features and advantages. AWS CDK is a nice combination between IaC and coding. Developers can build infrastructure without having to directly mess with static files.
There are so many other constructs you can work with, like: VPCs, EC2 instances, DynamoDB tables, and so on. I encourage you to continue exploring and hopefully this guide helps you to start building infrastructure with AWS CDK.
Your Turn
Can you create an S3 bucket with a different set of properties? Here’s a link to the Bucket construct to get you started.
Remember to start small, work your way up, and happy coding ππΎ!