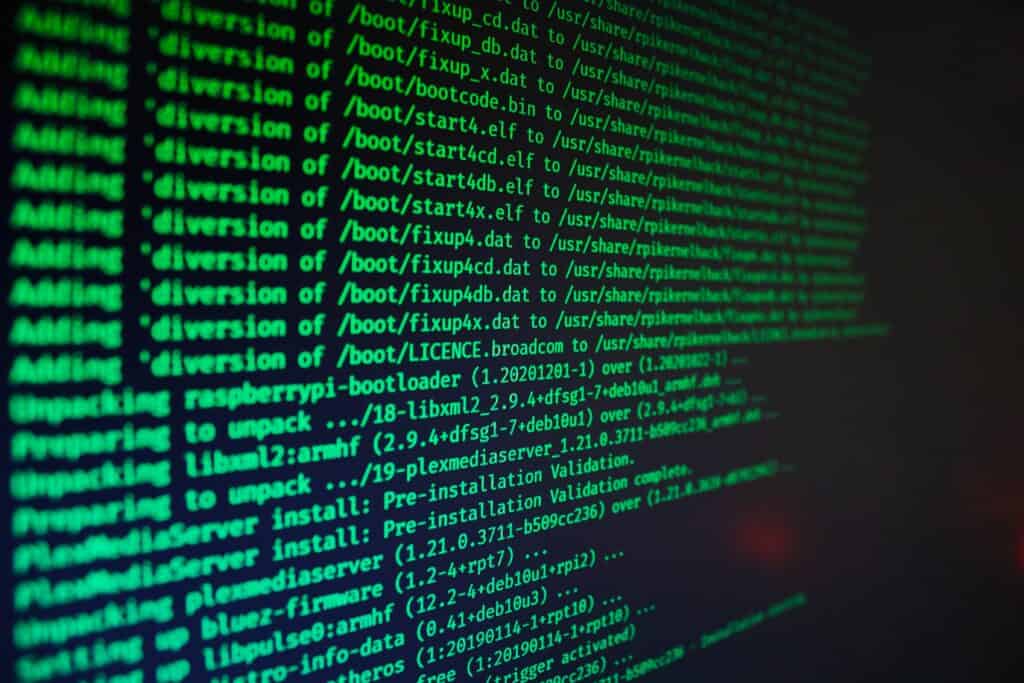
Similar to how natural languages can have a plethora of words, but we only use a subset of them, working with a command line interface is no different. Sure there are many commands (and you could even create your own), but there’s a subset that most developers use consistently.
- ls: list files in directory
- ls -a: list all files (including hidden files) in directory
- ls -l: list long format (show permissions)
- ls -la: combination of previous commands
- ls -S: list and sort by file size
- ls -t: list and sort by date-time
- ls -lah: list long format and shows size of file/directories in a human-readable format
ls -lh
is similar to du -h
. du
shows the disk usage aka how much space each file takes up, but it doesn’t show permissions, owner, or creation date like ls -lh
.
Example
user$ ls -la ~/Downloads/aFolder/
user$ du -h ~/Downloads/aFolder/
I’m always using ls
to see what’s in a directory; I quickly forget the names of files.
- history: displays a list of commands used during a terminal’s session
Example
user$ history
496 git status
497 git status
498 git add .
499 git commit -m "inital commit"
500 git push -u
501 cd Downloads/
This command is amazing, especially if you want to reuse a command from earlier but don’t want to hit the up arrow for several minutes.
To reuse a command from earlier, use the exclamation mark (!) with the index number.
!501
- cat: prints a file’s content onto the terminal
Example
user$ cat notes.txt
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Enim facilisis gravida neque convallis. Et odio pellentesque diam volutpat commodo sed egestas egestas fringilla. Sit amet facilisis magna etiam tempor. Id consectetur purus ut faucibus pulvinar elementum integer enim. Non curabitur gravida ar.
You can also use cat
to make a new file. This is an alternative to touch
or echo
.
user$ cat > newfile.txt
user$ touch newfile2.txt
user$ echo "" > newfile3.txt
If you only want the first 10 lines of a file, use head; if you want the last 10 lines of a file, use tail. You can also change how many lines are returned by using the -n
flag.
user$ head notes.txt
user$ tail notes.txt
user$ head -n 5 notes.txt
user$ tail -n 8 notes.txt
- clear: clears terminal screen
- man: returns helpful info about a command
Example
user$ man clear
clear(1) General Commands Manual clear(1)
NAME
clear - clear the terminal screen
SYNOPSIS
clear
DESCRIPTION
clear clears your screen if this is possible. It looks in the
environment for the terminal type and then in the terminfo database to
figure out how to clear the screen.
clear ignores any command-line parameters that may be present.
SEE ALSO
tput(1), terminfo(5)
This describes ncurses version 5.7 (patch 20081102).
clear(1)
~
~
(END)
:q
user$ clear
The clear command is handy when your terminal starts to look cluttered. I don’t know about you, but I get a bit claustrophobic when my terminal screen is littered with text. I like to start anew 😌.
- cd: change into a directory
- mkdir: make a new directory
- rm: delete a file
- rm -r: delete a directory that has files in it
- rmdir: delete an empty directory
Example
Documents user$ mkdir aFolder2
Documents user$ ls
aFolder
aFolder2
Bots!
CS1302 Lab04.ipynb
Documents user$ cd aFolder2
aFolder2 user$ cd ..
Documents user$ rmdir aFolder2
Documents user$ ls
aFolder
Bots!
CS1302 Lab04.ipynb
- chmod: change permissions of a file or directory
I usually use chmod +x file
to make a file executable.
user$ chmod +x example.jar
- grep: searches for a string in text
Example
(venv) user$ pip list | grep jupyter
jupyter 1.0.0
jupyter-client 6.1.12
jupyter-console 6.4.0
jupyter-core 4.7.1
jupyter-server 1.4.1
jupyterlab 3.1.4
jupyterlab-pygments 0.1.2
jupyterlab-server 2.6.2
jupyterlab-widgets 1.0.0
In the above example, I’m searching for the keyword jupyter
in my python packages. pip list
will generate a list of all the installed packages for this virtual environment, the pipe symbol |
will pass text (which is the list of installed packages), and grep
will filter on lines that contain jupyter
.
Hopefully, you’ve found some of these commands helpful. The more you use the command line, the more you’ll feel at ease when doing your daily tasks. Gaining confidence in using the CLI will help you become a better programmer.